Wednesday, February 4, 2015
Android beginner tutorial Part 12 EditText component
The EditText component is used to allow the user enter text. EditText is a class that extends TextView, but instead of just displaying the text, it displays it in a text field with a slight border and lets the user edit the text by tapping on the field.
The main class of the EditText class is getText(). It returns the text that is currently the value of this text field, but the type of the returned value is Editable, not String. The difference is that the Editable type is a text interface, which has variable text (based on the changes in the text field), as opposed to String, which is just a piece of text that doesnt change.
Theres also a method called setHint(), which displays a tip that is supposed to give the user an idea of what the text fields purpose is. Its value can be set to, for example, "Enter text here..." or "Your message..." The user sees it as the value of the textfield before he taps on it. Then it gets changed to whatever text he enters.
There are also a few methods in the class for managing text selection, such as selectAll(), setSelection(int start, int stop), setSelection(int index). The difference between the two setSelection() methods is that the first way to use it is to specify the beginning and ending indices of the selection, while the other way to use it is to just provide 1 value which sets the cursor to a specific index position.
Lets create a simple example in our activity_main.xml layout in our Android project.
Heres the code:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/textHint"
android:textSize="24sp"/>
</LinearLayout>
The strings.xml code:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">Code For Food Test</string>
<string name="textHint">Enter your message here!</string>
<string name="menu_settings">Settings</string>
</resources>
The results:
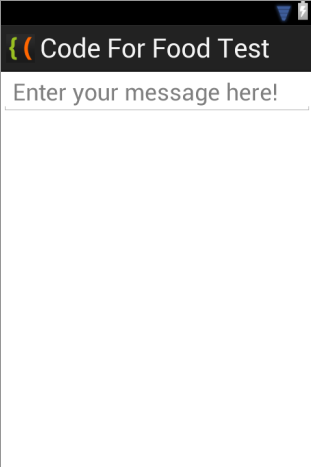
If you test your application now, youll see that after tapping on the text field, a keyboard UI pops up just like it usually does when entering text using the device.
Thats all for today.
Thanks for reading!
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.